1. Comments¶
1.1. Objectives¶
- Recognize and implement the three types of java style comments
1.2. Time Goal¶
- 15 minutes on this section
1.3. Key Terms¶
- comment
- Text a programmer adds to code, to be read by humans to better understand the code, but ignored by the compiler
- documentation
- Comments that describe the technical operation of code.
- inline comment
- A commment that appears after a statement on the same line.
- single-line comment
- In Java, starts with
//
and includes all the following text on that line. This is also known as an end-of-line comment or an inline comment. - multi-line comment
- In Java, starts with
/*
and ends with*/
, where all text between/*
and*/
is part of the comment. A multi-line comment is also known as a block comment. - JavaDoc comment
- In Java, a specially formatted multi-line comment that can be converted to program documentation in HTML (web page format).
- library
- A collection of resources used by computer programs
1.4. Exercises¶
-
Q-63: Which of the following are true about comments
- A comment can be plain english text that explains the code
- Correct
- When java sees
/*
it ignores all text until the end of the line - Incorrect
- You would never comment out actual code
- Incorrect, sometimes you may want to comment out code so that portion of the code is not compiled.
- Comments can make it easier for other programmers to understand what your code is meant to do
- Correct
- Comments can make it easier for you to understand the execution of code that you have written
- Correct
Note
You can extract documentation from your source code, and generate well-formatted HTML pages, using a tool called Javadoc. That way, you can write documentation as you go, and as things change, it is easier to keep the documentation consistent with the code. The Javadoc tool is included with the Java compiler, and it is widely used. In fact, the official documentation for the Java library (https://docs.oracle.com/javase/8/docs/api/) is generated by Javadoc. This can be a useful place to learn about code that has already been written that you can use in your programs.
Javadoc scans your source files looking for Javadoc comments. They begin with /**
(two stars) and end with */
(one star). Anything in between is considered part of the documentation.
Here’s a class definition with two Javadoc comments, one for the Goodbye class and one for the main method:
Run the code (triangular button above “Goodby.java”), and notice how the comments do not impact the execution of the program. The class comment explains the purpose of the class. The method comment explains what the method does.
Click the pencil icon next to the triangular run-button above to get back to the code. Notice that this example also has an inline comment //
. In general, these comments are short phrases that help explain complex parts of a program. They are intended for other programmers reading and maintaining the source code.
In contrast, Javadoc comments are longer, usually complete sentences. They explain what each method does, but they omit details about how the method works. And they are intended for people who will use the methods without looking at the source code.
Appropriate comments and documentation are essential for making source code readable. And remember that the person most likely to read your code in the future, and appreciate good documentation, is you.
Note
Javadoc tags
It’s generally a good idea to document each class and method, so that other programmers can understand what they do without having to read the code.
To organize the documentation into sections, Javadoc supports optional tags that begin with the at sign @
. For example, we can use @author
and @version
to provide information about the class. For example:
/**
* Utility class for extracting digits from integers.
*
* @author Chris Mayfield
* @version 1.0
*/ public class DigitUtil {
Documentation comments should begin with a description of the class or method, followed by the tags. These two sections are separated by a blank line (not counting the *
).
When we get to implementing our own methods, we will use @param
and @return
to provide information about parameters and return values. We will learn about what these actually mean soon, but knowing what a method’s parameters and return type is very useful for Java programming.
/**
* Tests whether x is a single digit integer.
*
* @param x the integer to test
* @return true if x has one digit, false otherwise
*/ public static boolean isSingleDigit(int x) {
The figure below shows part of the resulting HTML page generated by Javadoc. Notice the relationship between the Javadoc comment (in the source code) and the resulting documentation (in the HTML page).
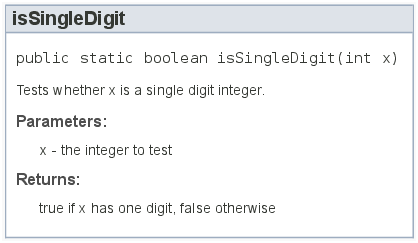
are used to document a program and improve its readability.
-
Q-64: Which of the following are true about comments?
/*
is the correct syntax for starting a Javadoc comment- Incorrect
- Comments do not cause any action to be performed during the program execution
- Correct
*/
is the correct syntax for ending a Javadoc comment- Correct
*/
is the correct syntax for ending a multi-line comment- Correct
/**
is the correct syntax for starting a multi-line comment- Incorrect
- No output
- Incorrect
- Input-output error for trying to print a comment
- Incorrect
This is a comment!
- Incorrect
"This is a comment!"
- Incorrect
// This is a comment!
- Correct
Q-65: What is the output of the following statement?
System.out.println("// This is a comment!");